Postgres JDBC is a freely available API that allows us to connect Java with the PostgreSQL database. You can download its JAR file or use its maven dependency to integrate PostgreSQL with Java. After this, you can connect the PostgreSQL database with Java and execute PostgreSQL queries from a Java application.
In this guide, we’ll show you a step-by-step implementation of connecting a PostgreSQL database with a Java application.
How to Connect PostgreSQL to Java Using JDBC
To connect PostgreSQL to Java via JDBC, simply follow the below-mentioned steps:
- Integrate PostgreSQL JDBC Driver with Java
- Connect PostgreSQL With Java
- Run PostgreSQL Queries From Java
Step1: Integrating Postgres JDBC Driver with Java Project
First, integrate the JDBC driver within a Java project. We can do this by integrating a JAR file or using a Maven dependency.
1) Using Maven Dependency
The most convenient way of integrating PostgreSQL to Java is by adding the Maven dependency:
<dependency> <groupId>org.postgresql</groupId> <artifactId>postgresql</artifactId> <version>42.7.3</version> </dependency>
2) Using JAR File
First, download the JDBC’s JAR file from PostgreSQL’s website. After this, create a Java application and embed the downloaded JAR file into your Java application. For this purpose, right-click on your Java application from the left pane and select the “Properties” option:
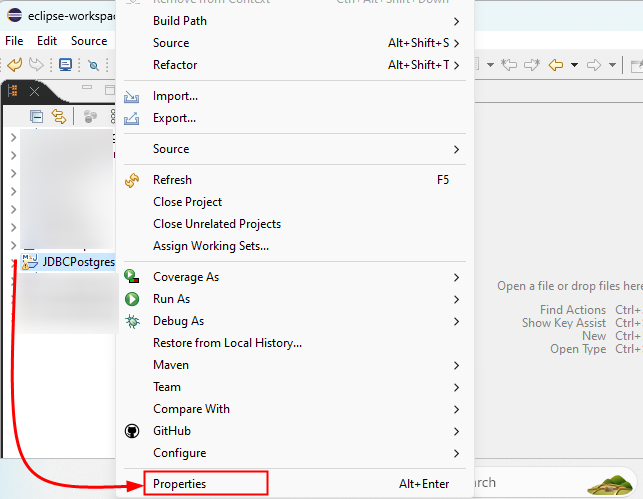
Now click on the “Libraries” tab and finally click on the “Add External JARs…” button. Upon clicking the “Add External JARs…” button, a new window pops up, select the downloaded JAR file, and click on the open button:
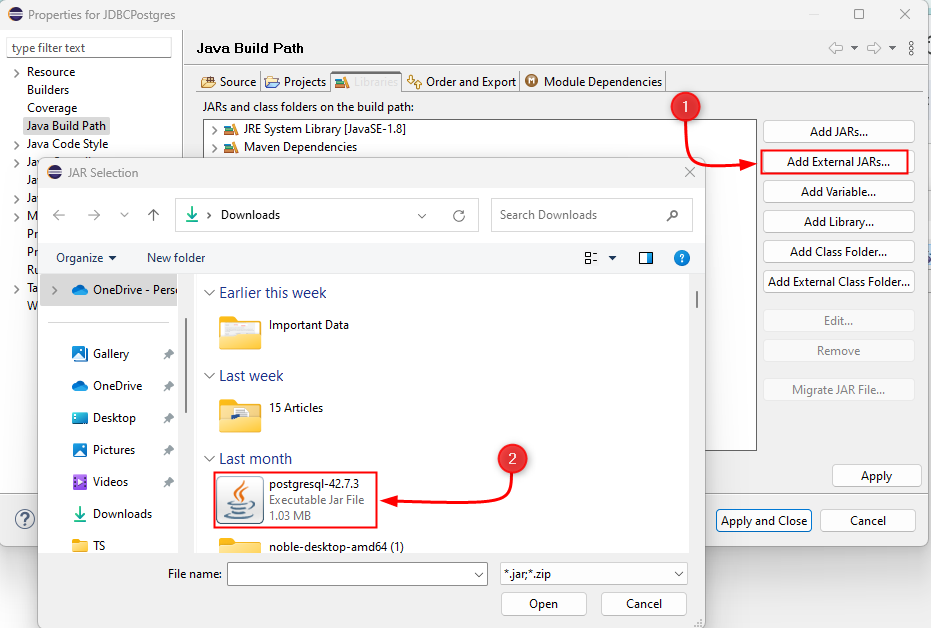
In the end, click on the “Apply and Close” button to complete the process:
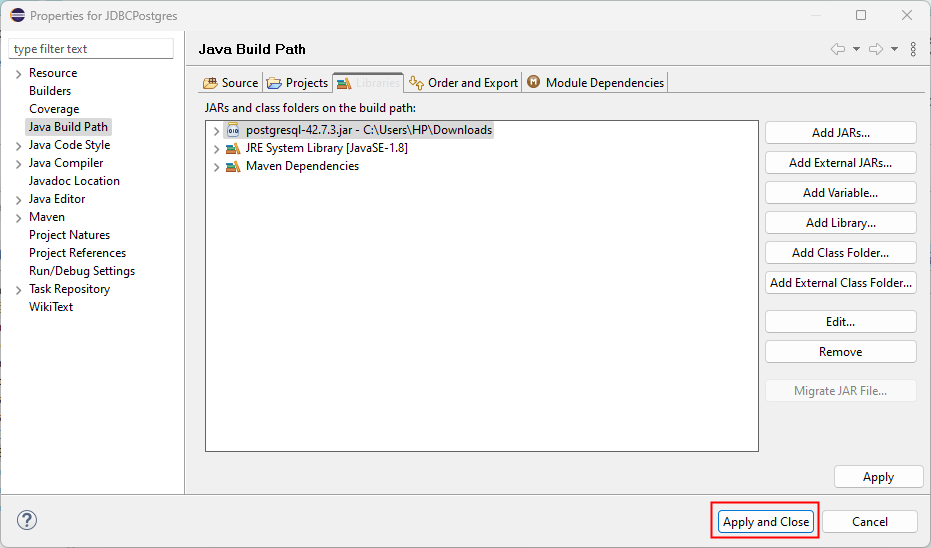
Step 2: Connect PostgreSQL With Java
Once PostgreSQL is integrated with Java, establish a connection between PostgreSQL and Java using the below code snippet:
import java.sql.*; public class PostgresJavaConnection { public static void main(String[] args) throws ClassNotFoundException, SQLException { String connect = "jdbc:postgresql://localhost:5432/postgres"; String user = "postgres"; String pwd = "postgres12345"; Class.forName("org.postgresql.Driver"); try (Connection conn = DriverManager.getConnection(connect, user, pwd)) { System.out.println("PostgreSQL has been Successfully Connected With Java!"); } catch (SQLException exc) { exc.printStackTrace(); } } }
In this code
- First, we import all classes/interfaces from the “java.sql” package.
- Next, we create string-type variables to specify a connection string, username, and password.
- After this, we use the “forName()” method to register the JDBC driver.
- Finally, we invoke the “getConnection()” method to establish a successful connection between Java and Postgres.
Output
The output confirms that a connection has been established between PostgreSQL and Java:

Step 3: Executing Select Query
Now we are all set to execute database query from Java. In this example, we’ll show you how to execute a SELECT query from Java:
Statement sql_statement = conn.createStatement(); ResultSet table = sqlStatement.executeQuery("SELECT * FROM bike_details"); while (table.next()) { String tableCol= table.getString("bike_number"); System.out.println("Bike Number = " + tableColumns); }
In this code,
- We invoke the “createStatement()” method to send SQL queries to the PostgreSQL database.
- After this, we call the executeQuery() method to run the SELECT query. As a result, the SELECT query will retrieve data from the bike_details table.
- After this, we wrap the “next()” method within the while loop to iterate each record of the “bike_details” table.
- In the end, we print each table record on the console:
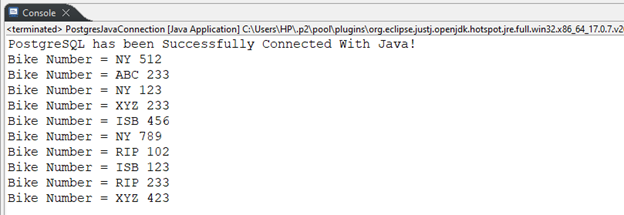
Step 4: Creating a New Table
In this example, we’ll show you how to create a new PostgreSQL table from Java:
Statement sql_statement = conn.createStatement(); String createNewTable = "CREATE TABLE cp_table (" + "cp_id serial PRIMARY KEY," + "cp_title TEXT," + "published_articles TEXT," + "cp_email VARCHAR(255))"; sql_statement.execute(createNewTable); System.out.println("Table Created!");
In this code,
- We execute an SQL statement “CREATE TABLE” to create a new table named “cp_table” with four columns.
- After this, we pass this SQL query as an argument to the execute() method to run this statement from Java.
- Finally, a message will be printed on the console stating “Table Created!”.

Similarly, we can run any PostgreSQL command/query from Java to execute several database operations.
Final Thoughts
JDBC is a freely available driver that lets us connect PostgreSQL with Java. To do this, we can download the JAR file of JDBC and add it to the build path of our Java project.
Alternatively, we can create a Maven project, and add the Postgres JDBC dependency in its pom.xml file. Doing this will integrate the Postgres database with Java programming. Next, we can invoke the getConnection() method to connect PostgreSQL with Java.
After this, we can execute any PostgreSQL query from Java to perform different database operations.