Postgres offers a built-in RANDOM() function that generates a random numeric value between “0(inclusive)” and “1(exclusive)”. In PostgreSQL, the RANDOM() function can be used to get a random number between a specific range. It doesn’t require any argument/parameter.
This post presents a comprehensive guide on how to generate random numbers in PostgreSQL.
How to Use RANDOM() Function to Get Random in Postgres?
The below-provided syntax is used in Postgres to get a random number between 0 and 1:
SELECT RANDOM();
Use the below-provided syntax to get a random number between a specific range:
SELECT RANDOM()*(Num_2- Num_1) + Num_1;
Where “Num_1” is the least value and “Num_2” represents the greatest value.
The return type of the RANDOM() function is DOUBLE PRECISION. However, the functions like FLOOR() and TRUNC() can be used with the RANDOM() function to get a random integer.
SELECT FLOOR(RANDOM()*(Num_2- Num_1 + 1)) + Num_1;
The above-given syntax will generate a random integer between Num_1 and Num_2, inclusive.
Example 1: Generating a Random Number
The following example demonstrates how to generate a random numeric value between 0(included) and 1(not included) via the RANDOM() function:
SELECT RANDOM() AS random_number;
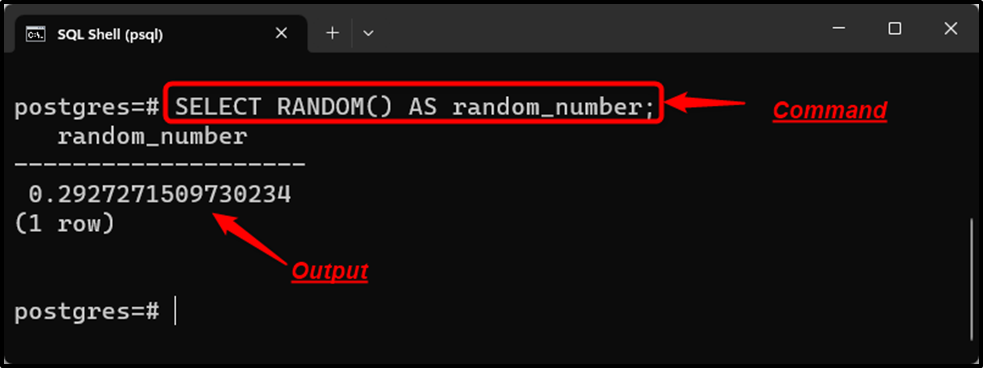
A random number between the range “0<=num < 1” has been generated successfully.
Example 2: Generating a Random Number Between Specific Range
The below snippet shows how to get a random numeric value between a specific range, let’s say “num >=5” and “num <15”:
SELECT RANDOM()*(15 - 5) + 5;
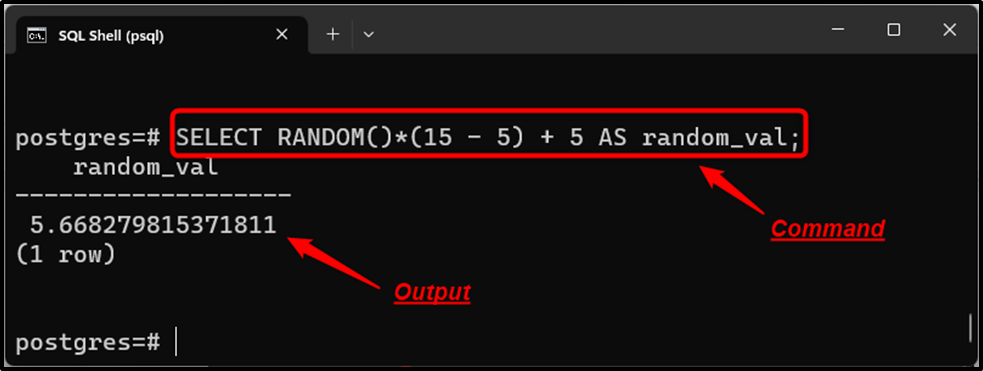
A random numeric value has been generated between the given range, i.e., “5<= num < 15”.
Example 3: Generating a Random Integer Between a Specific Range
The below-given code will generate a random integer between 5 and 25:
SELECT FLOOR(RANDOM()*(25 - 5 + 1)) + 5 As random_val;
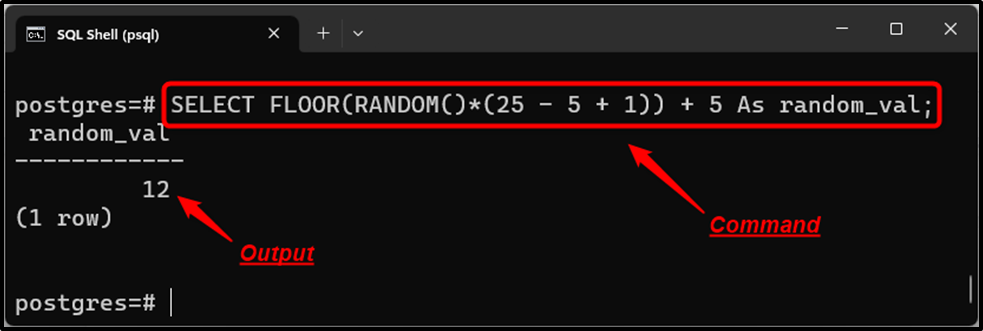
A random integer has been successfully generated between the specified range.
Example 4: Using RANDOM() Function on Table’s Data
In Postgres, the RANDOM() function can be used with the ORDER BY clause to get the random records of a particular table. For instance, the below snippet shows all rows of the “emp_data” table:
SELECT * FROM emp_data;
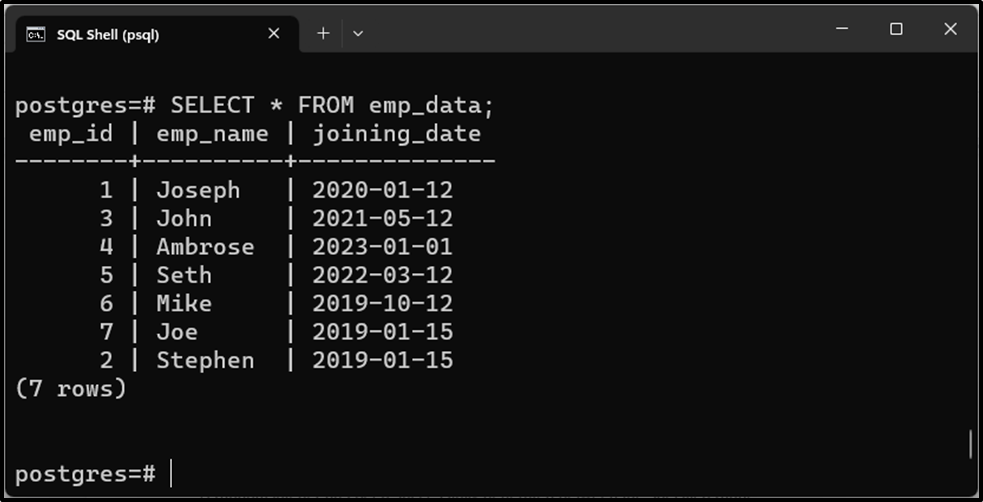
Use the RANDOM() function to get three random records from the selected table:
SELECT * FROM emp_data ORDER BY RANDOM() LIMIT 3;
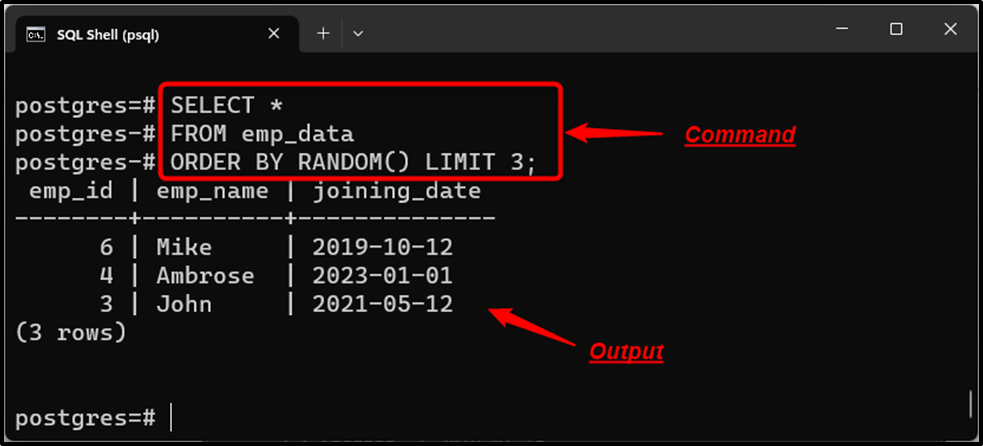
Three random records have been generated from the “emp_data” table.
Conclusion
In Postgres, the RANDOM() is an in-built function that generates a random numeric value between “0(inclusive)” and “1(exclusive)” or between a specific range. It doesn’t require any argument/parameter. The return type of the RANDOM() function is DOUBLE PRECISION. However, the functions like FLOOR() and TRUNC() can be used with the RANDOM() function to get a random integer. This post presented a comprehensive guide on how to generate random numbers in Postgres using the RANDOM() function.