Postgres offers several built-in functions and operators that are used to perform division on different numeric values. For instance, the “/” operator, DIV() function, MOD() function, etc. The return type of all these functions/operators depends on the data type of the given values.
This post will demonstrate all the possible ways to perform division on the given numbers in Postgres.
How to Use DIV(), MOD(), /, and % in Postgres?
The “/” operator and DIV() function perform the division on given numbers and retrieves the integer quotient. On the other hand, the MOD() function and “%” operator perform the division on the given numbers and retrieve the remainder instead of the quotient.
Let’s put these functions and operators into practice.
Example 1: A Comparative Analysis
In this example, we will use DIV() function, MOD() function, “/” operator, and the “%” operator side-by-side for a profound understanding:
SELECT DIV(550, 2) AS div_function, (550/2) AS Division_operator, MOD(550, 2) AS mod_function, (550%2) AS Remainder;
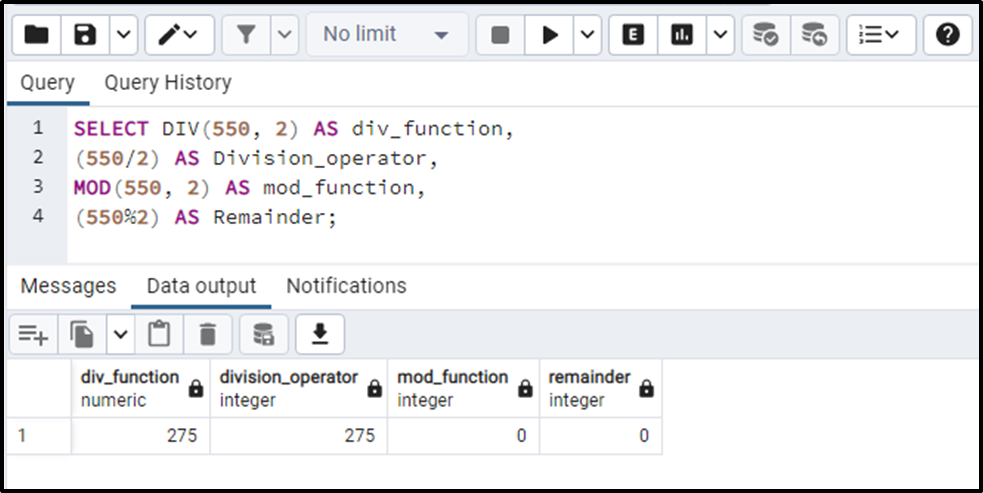
The output shows that the “DIV()” function and “/” operator retrieves the same result, i.e., quotient integer. On the other hand, the MOD() function and the “%” operator retrieves the same result, i.e., the remainder of two numbers.
Example 2: How to Perform Division in Postgres Using DIV() Function?
We have a sample table named “emp_data” that contains the following data:
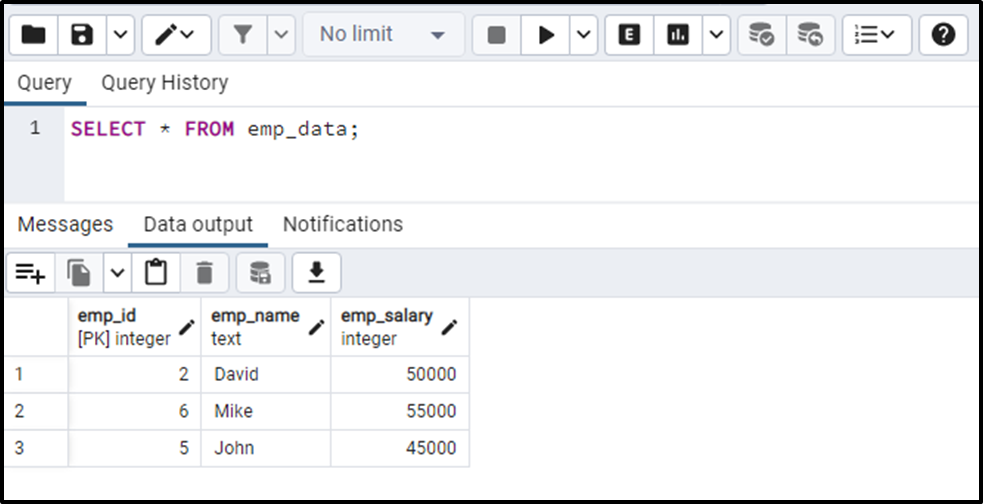
Suppose we have to find the half salary of each employee; for this purpose, we will use the DIV() function as follows:
SELECT emp_name, emp_salary, DIV(emp_salary, 2) AS half_salray FROM emp_data;
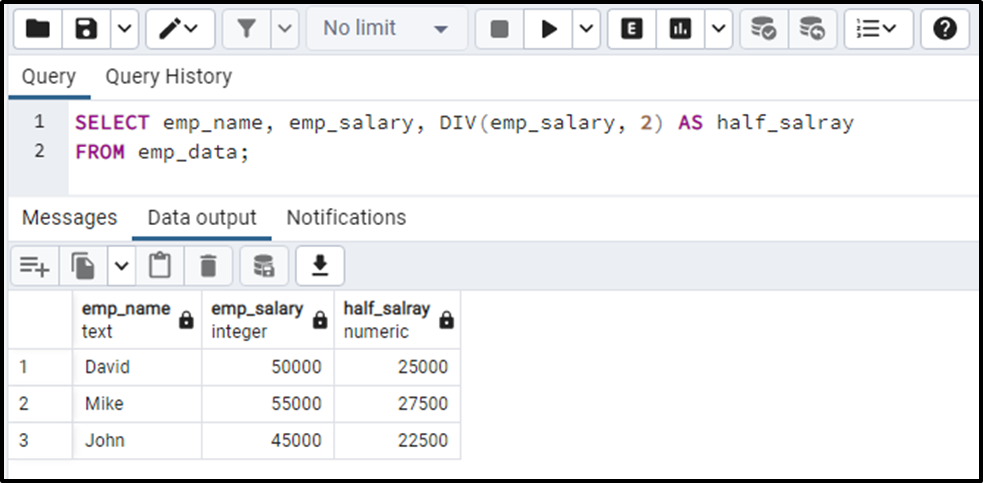
The output shows that the stated function successfully performs the division and retrieves the quotient.
Example 3: How to Perform Division in Postgres Using / Operator?
The below coding example will help you understand the usage of the “/” operator in a better way:
SELECT emp_name, emp_salary, (emp_salary/2) AS half_salray FROM emp_data;
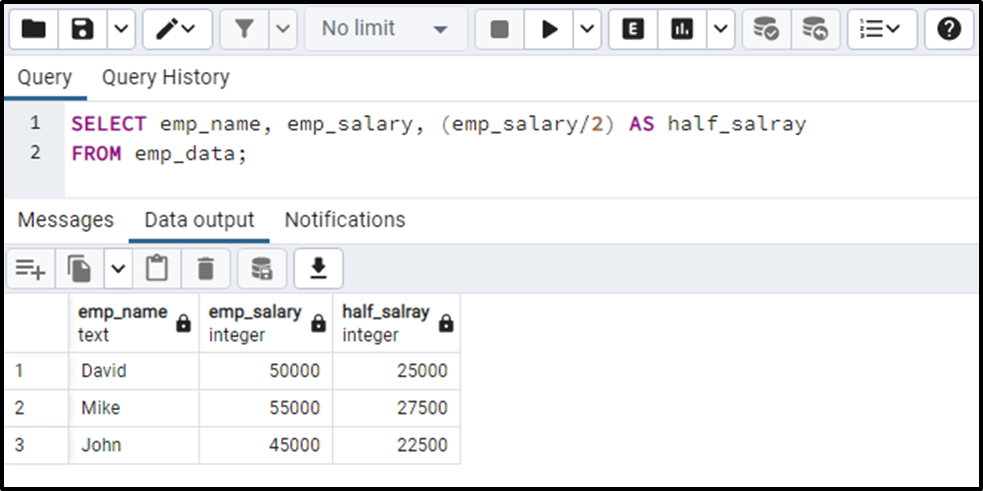
The output shows that the “/” operator retrieves the same results as the DIV() function.
Example 4: How to Perform Division in Postgres Using MOD() Function?
The below code illustrates how the MOD() function work in Postgres:
SELECT emp_name, emp_salary, MOD(emp_salary, 2) FROM emp_data;
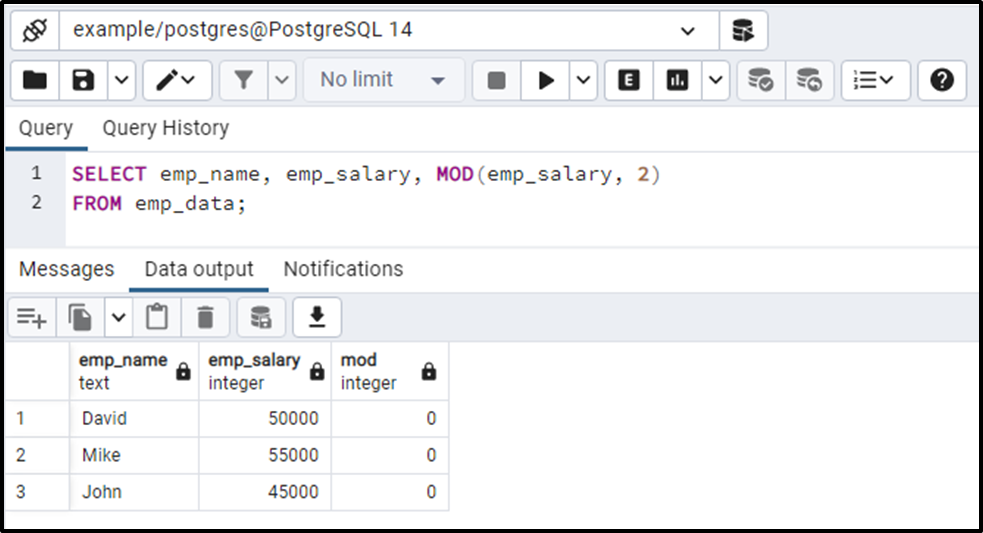
The output shows that the MOD() function retrieves the remainder instead of the quotient.
Example 5: How to Perform Division in Postgres Using % Operator?
The below coding example will help you understand this concept better:
SELECT emp_name, emp_salary, (emp_salary%2) AS REMAINDER FROM emp_data;
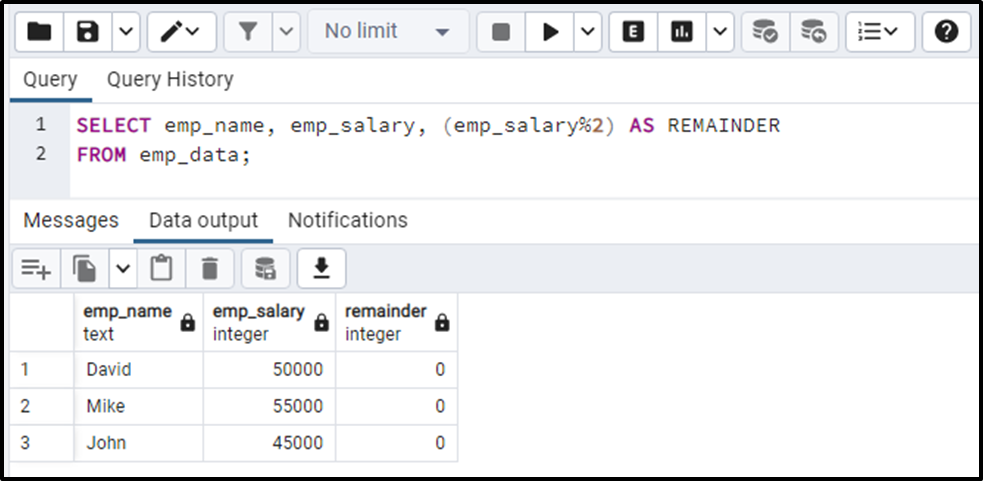
The output snippet signifies that the “%” operator generates the same results as the MOD() function.
Conclusion
Postgres offers several built-in functions and operators that are used to perform division on different numeric values. For instance, the “/” operator, DIV() function, MOD() function, etc. The “/” operator and DIV() function perform the division on given numbers and retrieves the integer quotient. On the other hand, the MOD() function and “%” operator perform the division on the given numbers and retrieve the remainder instead of the quotient. This post presented various examples of performing division on the given numbers in PostgreSQL.